SE456 Computer Vision
Assignment # 1
:Submitted By
Muhammad Taimur Adil, 110165097
Tuesday, September 10, 2013
Question 3
Write a matlab code to read the files owl.ppm in matrix mR1, mG1 and mB1.
Similarly, read the file mecca06.ppm in matrix mR2, mG2 and mB2. Write these
matrices in the files with the following names.
owl.ppm should be written as owl_Out.ppm
mecca06.ppm should be written as mecca06_Out.ppm
Code
IM1 = imread('Z:\CV\Images\owl.ppm');
IM2 = imread('Z:\CV\Images\mecca06.ppm');
mR1=IM1(:,:,1);
mG1=IM1(:,:,2);
mB1=IM1(:,:,3);
mR2=IM2(:,:,1);
mG2=IM2(:,:,2);
mB2=IM2(:,:,3);
figure
imshow(IM1);
figure
imshow(IM2);
imwrite(IM1,'Z:\CV\output images\owl_Out.ppm');
imwrite(IM2,'Z:\CV\output images\mecca06_Out.ppm');
Description of Code
In above code i am just read original colored image with extension ppm and save them with different name.
Result
It just read and display the original image so there will be no change.
Input Images
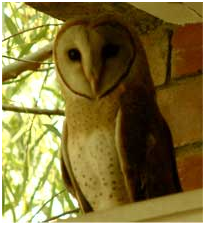
Output Image
Question 4
Write a MATLAB program that do the following:
a) Reads the files (owl.ppm and mecca06.ppm) in a matrix mR1, mG1, mB1,mR2, mG2, mB2 (in general m means matrix, R means Red layer, G means green layer, B means Blue layer, 1 means first image i.e., owl.ppm and 2 means second image i.e., mecca06.ppm)
b) Make each pixel of the G matrix (matrix containing green layer) and B matrix of both the images to 0 (i.e., make the matrices m1G, m1B, m2G and m2B to zero). Write these three matrices (of red, green and blue layer) of each image ina file named owl_Red_out.ppm
c) Make each pixel of the R matrix (matrix containing red layer) and B matrix ofboth the images to 0 (i.e., make the matrices m1R, m1B, m2R and m2B to zero). Write these three matrices (of red, green and blue layer) of each image in a file named owl_Green_out.ppm
d) Make each pixel of the R matrix (matrix containing red layer) and G matrix ofboth the images to 0 (i.e., make the matrices m1R, m1G, m2R and m2G to zero). Write these three matrices (of red, green and blue layer) of each image in a file named owl_Blue_out.ppm
e) Make each pixel of the R matrix (matrix containing red layer) to zero. Writethese three matrices (of red, green and blue layer) of each image in a file named owl_GreenBlue_out.ppm
f) Make each pixel of the G matrix (matrix containing green layer) to zero. Writethese three matrices (of red, green and blue layer) of each image in a file named owl_RedBlue_out.ppm
g) Make each pixel of the B matrix (matrix containing blue layer) to zero. Write these three matrices (of red, green and blue layer) of each image in a file named owl_RedGreen_out.ppm
h) Take the logarithm (log) of base 10 of each pixel of the three (red, green andblue) matrices of the images and save the matrices in the files (owl_Log_Out.ppm and mecca06_Log_Out.ppm)
i) Generate the gray scale image of these colored images i.e., owl.ppm and mecca.ppm. Take the average of pixels of these three matrices of each image and generate one matrix. Later save this matrix in a file named owl_grayScale_Out.ppm and mecca06_grayScale_Out.ppm
Code
%part A -------------------------------------------------------------------
% Reads the files (owl.ppm and mecca06.ppm) in a matrix mR1, mG1, mB1,
% mR2, mG2, mB2
img1=imread('Z:\CV\images\mecca06.ppm');
img2=imread('Z:\CV\images\owl.ppm');
mR1=img1(:,:,1);
mG1=img1(:,:,2);
mB1=img1(:,:,3);
mR2=img2(:,:,1);
mG2=img2(:,:,2);
mB2=img2(:,:,3);
figure
imshow(mR1);
figure
imshow(mG1);
figure
imshow(mB1);
figure
imshow(mR2);
figure
imshow(mG2);
figure
imshow(mB2);
imwrite(img1,'Z:\CV\output images ppm\mecca06_ Out.ppm');
imwrite(img2,'Z:\CV\output images ppm\owl_Out.ppm');
%part B -------------------------------------------------------------------
% Make red output
img1=imread ('Z:\CV\images\mecca06.ppm');
img2=imread('Z:\CV\images\owl.ppm');
img1(:,:,2)=0;
img1(:,:,3)=0;
img2(:,:,2)=0;
img2(:,:,3)=0;
figure
imshow(img1);
figure
imshow(img2);
imwrite(img1,'Z:\CV\output images ppm\mecca06_Red_Out.ppm');
imwrite(img2,'Z:\CV\output images ppm\owl_Red_Out.ppm');
%part C -------------------------------------------------------------------
% Make green output
img1=imread('Z:\CV\images\mecca06.ppm');
img2=imread('Z:\CV\images\owl.ppm');
img1(:,:,1)=0;
img1(:,:,3)=0;
img2(:,:,1)=0;
img2(:,:,3)=0;
figure
imshow(img1);
figure
imshow(img1);
imwrite(img1,'Z:\CV\output images ppm\mecca06_Green_Out.ppm');
imwrite(img2,'Z:\CV\output images ppm\owl_Green_Out.ppm');
%part D -------------------------------------------------------------------
% Make blue output
img1=imread('Z:\CV\images\mecca06.ppm');
img2=imread('Z:\CV\images\owl.ppm');
img1(:,:,1)=0;
img1(:,:,2)=0;
img2(:,:,1)=0;
img2(:,:,2)=0;
figure
imshow(img1);
figure
imshow(img2);
imwrite(img1,'Z:\CV\output images ppm\mecca06_Blue_Out.ppm');
imwrite(img2,'Z:\CV\output images ppm\owl_Blue_Out.ppm');
%part E -------------------------------------------------------------------
% Make green+blue output
img1=imread('Z:\CV\images\mecca06.ppm');
img2=imread('Z:\CV\images\owl.ppm');
img1(:,:,1)=0;
img2(:,:,1)=0;
figure
imshow(img1);
figure
imshow(img2);
imwrite(img1,'Z:\CV\output images ppm\mecca06_GreenBlue_Out.ppm');
imwrite(img2,'Z:\CV\output images ppm\owl_GreenBlue_Out.ppm');
%part F -------------------------------------------------------------------
% Make red+blue output
img1=imread ('Z:\CV\images\mecca06.ppm');
img2=imread('Z:\CV\images\owl.ppm');
img1(:,:,2)=0;
img2(:,:,2)=0;
figure
imshow(img1);
figure
imshow(img2);
imwrite(img1,'Z:\CV\output images ppm\mecca06_RedBlue_Out.ppm');
imwrite(img2,'Z:\CV\output images ppm\owl_RedBlue_Out.ppm');
%part G -------------------------------------------------------------------
% Make red+green output
img1=imread ('Z:\CV\images\mecca06.ppm');
img2=imread('Z:\CV\images\owl.ppm');
img1(:,:,3)=0;
img2(:,:,3)=0;
figure
imshow(img1);
figure
imshow(img2);
imwrite(img1,'Z:\CV\output images ppm\mecca06_RedGreen_Out.ppm');
imwrite(img2,'Z:\CV\output images ppm\owl_RedGreen_Out.ppm');
%part H -------------------------------------------------------------------
% take log of base 10 of each ppm image
C=imread('Z:\CV\images\mecca06.ppm');
D=imread('Z:\CV\images\owl.ppm');
img1=LOG10(double(C));
img2=LOG10(double(D));
figure
imshow(img1);
figure
imshow(img2);
imwrite(img1,'Z:\CV\output images ppm\mecca06_log_Out.ppm');
imwrite(img2,'Z:\CV\output images ppm\owl_log_Out.ppm');
%part I -------------------------------------------------------------------
% take the matrix red blue and green and then avg and make pgm image
img1=imread('Z:\CV\images\mecca06.ppm');
img2=imread('Z:\CV\images\owl.ppm');
mR1=img1(:,:,1);
mG1=img1(:,:,2);
mB1=img1(:,:,3);
mR2=img2(:,:,1);
mG2=img2(:,:,2);
mB2=img2(:,:,3);
A=(mR1+mG1+mB1)./3;
B=(mR2+mG2+mB2)./3;
figure
imshow(A);
figure
imshow(B);
imwrite(A,'Z:\CV\output images ppm\mecca06_grayScale_Out.pgm');
imwrite(B,'Z:\CV\output images ppm\owl_grayScale_Out.pgm');
Description of Code
- a) I use a function to read file in MATLABand store into a variable than take three matrix mR1, mG1 and mB1 and three matrix for other image and store red layer in mR1, green layer in mG1 and blue layer in mB1 and repeat it for second image. And at last save both images with different name.
- b) I read both images, as we know colored image is a combination of 3 layers red, blue and green so i remove green and blue layer with assigning them 0 so i got only one layer that is red so that i show it on screen and also save the image with different name.
- c) I read images, as we know colored image is a combination of 3 layers red, blue and green so i remove red and blue layer with assigning them 0 so i got only one layer that is green so that i show it on screen and also save the image with different name. I did this work with both colored images.
- d) I read images, as we know colored image is a combination of 3 layers red, blue and green so i remove green and red layer with assigning them 0 so i got only one layer that is blue so that i show it on screen and also save the image with different name.I did this work with both colored images.
- e) I read images, as we know colored image is a combination of 3 layers red, blue and green so i remove red layer with assigning 0 to red layer. So, I got two layers that are blue and green. So, that i shows it on screen and also save the image with different name.I did this work with both colored images.
- f) I read images, as we know colored image is a combination of 3 layers red, blue and green so i remove green layer with assigning 0 to green layer. So, I got two layers that are blue and red. So, that i shows it on screen and also save the image with different name.I did this work with both colored images.
- g) I read images, as we know colored image is a combination of 3 layers red, blue and green so i remove blue layer with assigning 0 to blue layer. So, I got two layers that are red and green. So, that i shows it on screen and also save the image with different name.I did this work with both colored images.
- h) In this part first I read both images take log10 of each colored image as we know in MATLAB log10 cannot be taken of the image that have uint8 format so I convert it to double and then take log10. After that I show the images and also save them with different name.
- i) In this part I have to sum the value of three matrix (As we know colored image has three layers red blue and green)and divide on 3 to take its average. After that I save it in A and B matrix. Then I show them on screen and save both image with different name.
Result
- a) Part (a) has no result because I have just save the ppm images with name mecca06_out and owl_out
- b) I have just removed the green and blue layer so we have only layer so our output image will be totally red.
- c) In this part I have just remove the two layers red and blue of the images. So, our output is totally green.
- d) In this part I have just remove the two layers red and green of the images. So, our output is totally blue.
- e) In this part I have just remove one layer that is red of the images. So, our output is greenish blue.
- f) In this part I have just remove one layer that is green of the images. So, our output images are reddish blue.
- g) In this part I have just remove one layer that is blue of the images. So, our output images are reddish green.
- h) It’s an important part because we take log10 of the colored images so due to log10 of every pixel value we get an image that contain three different color that has been seen by bare eyed (see Part H Output image).
- i) In this part we got an amazing output every pixel is the average value of the tree layer of color image. And we got grey image. I.e. Blue layer has 150 amount, Green layer has 52 amount and Red layer has 85 amount on its first index after processing we got a resultant matrix which has (85+52+150)/3 value that is 95.66 that is 96 approx. Because its only one layer so we got grey image.
Input Images
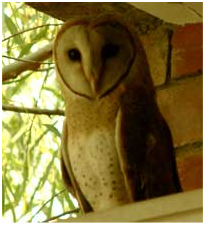
Output Image
Part A output images (Write with different name)
Part B output images (Make Red Output)
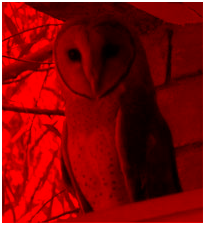
Part C output images (Make Green Output)
Part D output images (Make Blue Output)
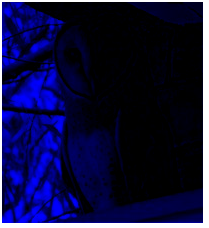
Part E output images (Make Green+Blue Output)
Part F output images (Make Red+Blue Output)
Part G output images (Make Red+Green Output)
Part H output images (Take log10)
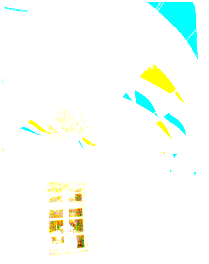
Part I output images (Make GreyScale of Colored Images)
0 comments:
Post a Comment